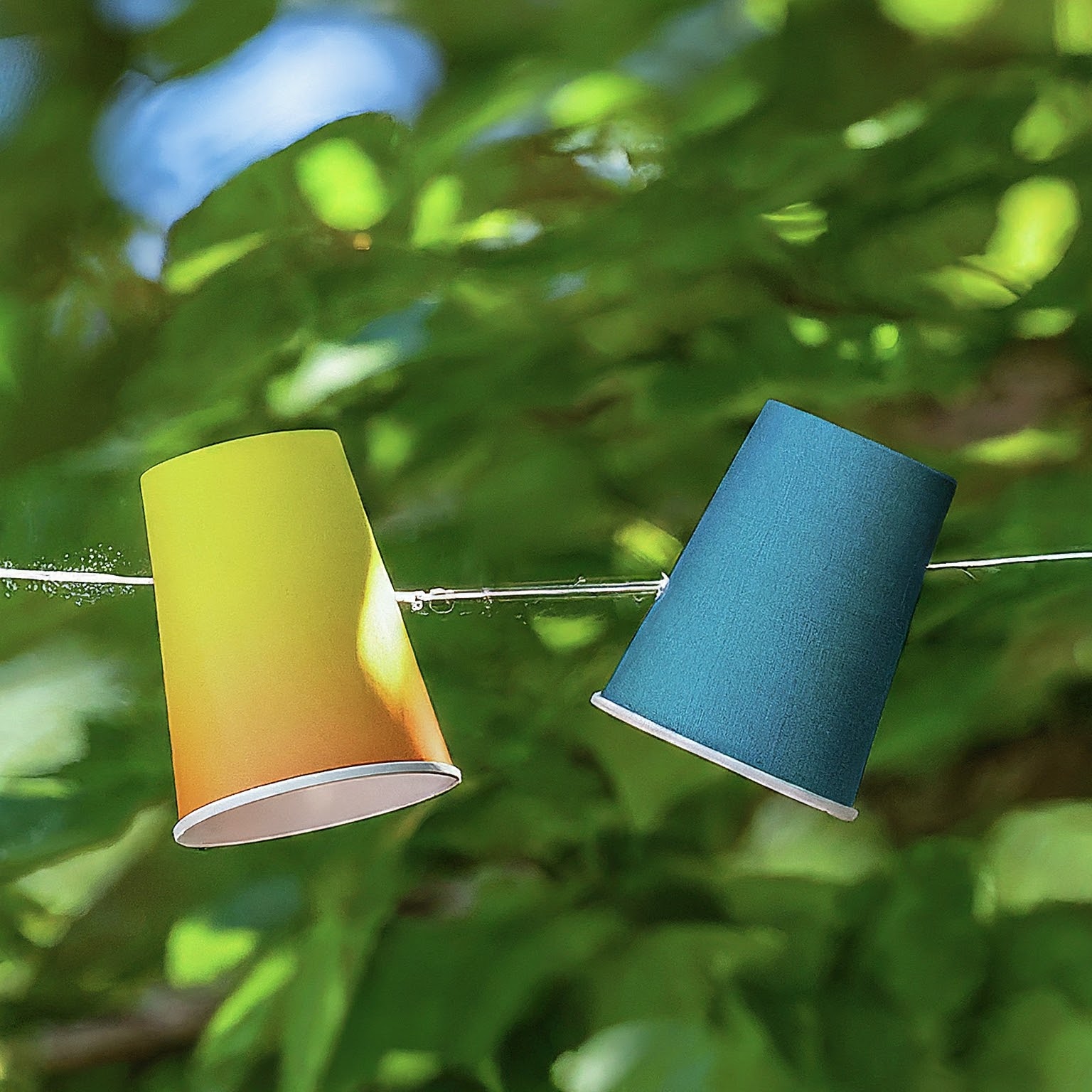
Exploring the Differences Between WebRTC Data Channels and WebSockets
- 5 minsIntroduction
WebRTC (Web Real-Time Communication) data channels and WebSockets are both powerful technologies that enable real-time communication between clients and servers (or other clients) to enable transfer of arbitrary data. However, they have distinct characteristics and use cases. In this blog post, we’ll delve into the key differences between WebRTC data channels and WebSockets, providing code snippets for better understanding.
WebRTC Data Channels
-
Purpose: WebRTC data channels are specifically designed for peer-to-peer communication between browsers. They are a part of the larger WebRTC framework, primarily used for real-time data exchange.
-
Peer-to-Peer Nature: WebRTC data channels establish a direct connection between peers, allowing for efficient and low-latency communication without the need for a centralized server.
-
Browser Support: WebRTC data channels are supported by modern browsers, making them suitable for applications that require real-time communication, such as remote desktop apps, online gaming, real time text chats, collaborative editing or file transfer.
Creating a WebRTC Data Channel:
// Creating a WebRTC connection
const peerConnection = new RTCPeerConnection();
// Creating a data channel
const dataChannel = peerConnection.createDataChannel("myDataChannel");
// Event handler for data channel messages
dataChannel.onmessage = (event) => {
console.log("Received message:", event.data);
};
// Event handler for data channel open
dataChannel.onopen = () => {
console.log("Data channel opened!");
};
// Event handler for data channel close
dataChannel.onclose = () => {
console.log("Data channel closed!");
};
WebSockets
-
Purpose: WebSockets are a general-purpose communication protocol that provides full-duplex communication channels over a single, long-lived connection. They can be used for various real-time applications beyond browsers.
-
Centralized Communication: Unlike WebRTC data channels, WebSockets typically rely on a centralized server to facilitate communication between clients. The server acts as an intermediary for message exchange.
-
Wide Range of Applications: WebSockets are widely used in applications like chat applications, financial trading platforms, live sports updates, and more. They provide a reliable and efficient means of bidirectional communication.
Establishing a WebSocket Connection in the browser
// Creating a WebSocket connection
const socket = new WebSocket("wss://example.com/socket");
// Event handler for connection open
socket.onopen = () => {
console.log("WebSocket connection opened!");
};
// Event handler for received messages
socket.onmessage = (event) => {
console.log("Received message:", event.data);
};
// Event handler for connection close
socket.onclose = () => {
console.log("WebSocket connection closed!");
};
Choosing Between WebSocket and WebRTC Data Channels
- Choose WebSocket When:
-
Centralized Communication: If your application involves a centralized server that facilitates communication between clients, WebSocket is a suitable choice. Examples include chat applications, real-time notifications, or online gaming where a central server manages interactions.
-
Broader Browser Support: WebSocket enjoys broader browser support, making it a more compatible choice for a wider range of scenarios, especially when cross-browser compatibility is crucial.
-
Simplicity and Ease of Implementation: WebSocket provides a straightforward API and is easier to implement for applications that do not require direct peer-to-peer communication.
-
- Choose WebRTC Data Channels When:
-
Peer-to-Peer Communication: If your application requires direct communication between browsers without relying on a central server, WebRTC Data Channel is ideal. This is particularly useful for scenarios like video conferencing, collaborative tools, or file sharing where low-latency, peer-to-peer communication is crucial.
-
Real-Time Media Streaming: When dealing with real-time media streams, such as audio or video, WebRTC is well-suited. The integrated support for media streams, along with the Data Channel for additional data transfer, makes it a comprehensive choice for applications that involve both.
-
Enhanced Privacy and Security: WebRTC inherently supports end-to-end encryption, providing an added layer of security for peer-to-peer communication. If privacy and security are paramount, especially in applications like video conferencing, WebRTC is a preferred choice.
-
- Considerations for Both:
-
Network Topology: Consider the network topology of your application. If a centralized server is already a fundamental part of your architecture, WebSocket may align better. For decentralized or peer-centric architectures, WebRTC Data Channel is more suitable.
-
Complexity and Development Time: WebSocket might be a quicker and simpler option for straightforward applications, while WebRTC can offer more advanced features but may require additional development effort, especially when dealing with complex scenarios like NAT traversal.
-
Scalability: WebSocket can be easier to scale since the central server can manage communication. WebRTC, being peer-to-peer, may require additional considerations for scaling, especially in large applications.
-
Ultimately, the choice between WebSocket and WebRTC Data Channel depends on the specific requirements and architecture of your application. Evaluate factors such as communication model, latency needs, privacy concerns, and development complexity to make an informed decision.
Conclusion
In summary, WebRTC data channels are ideal for peer-to-peer communication within browsers, while WebSockets offer a more general-purpose solution for real-time communication with the help of a centralized server. The choice between them depends on the specific requirements and use cases of your application. Understanding their differences will empower you to make informed decisions when implementing real-time communication features.