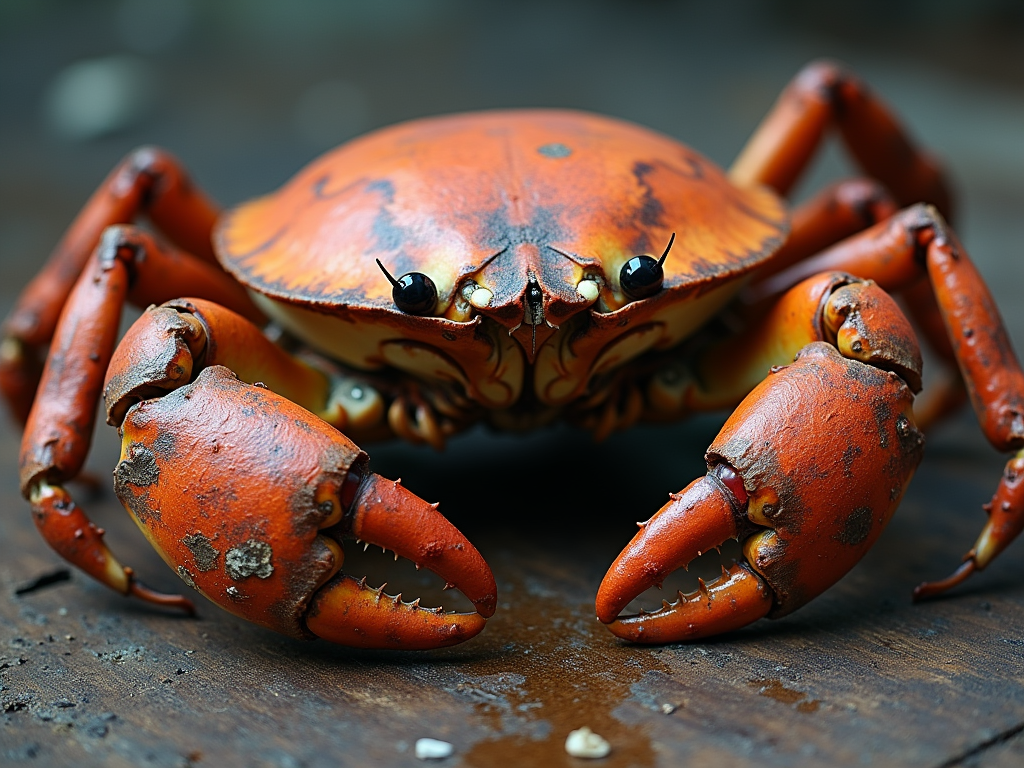
The Quest for Compile-Time String Formatting in Rust
- 4 minsImagine you’re deep in Rust code, building a CLI tool that’s going to make waves in the developer community. You’ve got your clap
derive macros set up perfectly, ready to handle command parsing with ease. But then you hit a snag. You need to concatenate some constants for your about
attribute to show compatibility information, and Rust isn’t playing ball.
“Hmm, compile-time string formatting is more complicated than I expected,” you think, furrowing your brow at the error message on your screen.
Welcome to Rust, where safety and zero-cost abstractions reign supreme, but sometimes the simplest tasks can become unexpected challenges.
The Problem: Rust’s Compile-Time Conundrum
Why can’t Rust handle simple string concatenation at compile time? Well, it’s not as straightforward as you might think.
Let’s look at an example that seems like it should work, but doesn’t:
use clap::Parser;
const TOOL_NAME: &str = "SuperTool";
const COMPATIBLE_CRATE: &str = "AwesomeDependency";
const COMPATIBLE_VERSION: &str = "1.2.3";
// This doesn't work!
const ABOUT: String = format!(
"{} - Compatible with {} version {}",
TOOL_NAME, COMPATIBLE_CRATE, COMPATIBLE_VERSION
);
#[derive(Parser, Debug)]
#[command(author = "Alice", version, about = ABOUT)]
struct Cli {
// CLI arguments would go here
}
fn main() {
let args = Cli::parse();
println!("{:?}", args);
}
Try to compile this, and Rust responds with an error:
error[E0015]: calls in constants are limited to constant functions, tuple structs and tuple variants
--> src/main.rs:8:21
|
8 | const ABOUT: String = format!(
| ^^^^^^ ...
error: aborting due to previous error
The format!
macro, usually so helpful for string formatting, suddenly becomes unavailable in the compile-time context.
Rust’s const evaluation capabilities, while continuously improving, still have limitations. The language designers have prioritized other features, leaving compile-time string formatting as a challenge to overcome.
The Solution: Leveraging Crates
Fortunately, the Rust ecosystem provides solutions. One such solution is the const_format
crate, which allows for compile-time string formatting.
With const_format
, you can write:
use const_format::formatcp;
const TOOL_NAME: &str = "SuperTool";
const COMPATIBLE_CRATE: &str = "AwesomeDependency";
const COMPATIBLE_VERSION: &str = "1.2.3";
const ABOUT: &str = formatcp!(
"{} - Compatible with {} version {}",
TOOL_NAME, COMPATIBLE_CRATE, COMPATIBLE_VERSION
);
This approach gives us the compile-time string formatting we need.
An Alternative: Build Scripts
There’s another way to achieve compile-time string formatting without additional crates: build scripts and environment variables.
By using a build.rs
file, we can generate our formatted strings at compile time and pass them to our main code as environment variables. Here’s how:
- Create a
build.rs
file in your project root:
fn main() {
let tool_name = "SuperTool";
let compatible_crate = "AwesomeDependency";
let compatible_version = "1.2.3";
let about = format!(
"{} - Compatible with {} version {}",
tool_name, compatible_crate, compatible_version
);
println!("cargo:rustc-env=ABOUT={}", about);
}
- In your
main.rs
, use the environment variable:
use clap::Parser;
#[derive(Parser, Debug)]
#[command(author = "Alice", version, about = env!("ABOUT"))]
struct Cli {
// CLI arguments would go here
}
fn main() {
let args = Cli::parse();
println!("{:?}", args);
}
This method allows us to perform the string formatting during the build process and inject the result into our main code.
Lessons Learned
This journey through compile-time string formatting in Rust teaches us a few valuable lessons:
- Rust prioritizes safety and performance, sometimes at the cost of convenience.
- The Rust ecosystem often provides solutions for language limitations.
- Build scripts are powerful tools that can help overcome certain restrictions.
- Sometimes, the simplest solutions (like using environment variables) can solve complex problems.
- With some creativity, we can usually find a way around language limitations.
While Rust may not support compile-time formatting out of the box, we have multiple ways to achieve our goals. Whether it’s through community-created crates or clever use of build scripts, Rust developers always find a way forward.